Billing customers
With the Usage API, you can determine which entities and events are billable each month.
As you grow your payroll business on top of Check’s platform, it's critical to understand customer usage of it to inform direct billing or adjacent invoicing applications. This guide provides an overview of how to leverage Check’s Usage API to support the realization of revenue. This API can be used to:
- Understand which companies, employees, and contractors should be billable in a month.
- Inform who should be billed for pass-through fees generated from failed fundings or wires.
- Help assess the profitability of individual customers and the overall customer base.
The Usage API has two core concepts:
UsageSummary
: provides summary-level usage statistics. By default, this API will list all statistics for all companies, and supports a filter to view the summaries for a single company. This endpoint lists usage counts of billable companies, employees, and contractors, along with associated fee-generating events such as wires and more.
{
"company": "com_dXE2p4wCM251HmlJB0Mn",
"category": "company",
"count": 1,
"period_start": "2024-05-01",
"period_end": "2024-05-31"
}
UsageRecord
: provides specific usage-level data for a given category (e.g., wire
), so you can break down the summary-level counts down to each instance of the usage event. This can be useful information to provide your customers when billing them.
{
"category": "wire",
"company": "com_dXE2p4wCM251HmlJB0Mn",
"resource_type": "payment_attempt",
"resource": "pyt_vIFHhvyGN47ej1upyIT8",
"effective_at": "2024-05-01T00:00:00.000Z"
}
Note, all times are in UTC.
Getting a usage summary
It's June, and company com_dXE2p4wCM251HmlJB0Mn
has paid 15 employees and 8 contractors in the month of May. During that month, the company had insufficient funds for a payroll so they generated one company ACH return. When the company was paying two of their employees, the payments went to closed accounts, causing 2 payee returns. If you retrieved UsageSummary
objects from the API filtered for that company for the month of May, you would get:
{
"previous": null,
"next": null,
"results": [
{
"company": "com_dXE2p4wCM251HmlJB0Mn",
"category": "company",
"count": 1,
"period_start": "2024-05-01",
"period_end": "2024-05-31"
},
{
"company": "com_dXE2p4wCM251HmlJB0Mn",
"category": "employee",
"count": 15,
"period_start": "2024-05-01",
"period_end": "2024-05-31"
},
{
"company": "com_dXE2p4wCM251HmlJB0Mn",
"category": "contractor",
"count": 8,
"period_start": "2024-05-01",
"period_end": "2024-05-31"
},
{
"company": "com_dXE2p4wCM251HmlJB0Mn",
"category": "company_funding_failure",
"count": 1,
"period_start": "2024-05-01",
"period_end": "2024-05-31"
},
{
"company": "com_dXE2p4wCM251HmlJB0Mn",
"category": "payee_failed_payment",
"count": 2,
"period_start": "2024-05-01",
"period_end": "2024-05-31"
}
]
}
With this information, you can generate the total bill for this given company, annotating it with the pricing data that lives in your application.
Categories
category | Description |
---|---|
company | Billable company. |
employee | Billable employee. |
contractor | Billable contractor. |
company_funding_failure | Funding failure for company, originating from insufficient funds or account closure, for example. |
payee_failed_payment | The first failed payment to a payee's bank account, originating from a failed bank account verification or account closure, for example. |
wire | Payroll funded by wire when there is no company_funding_failure |
one_day_payroll_processing_period | Payees that have used the one day payroll processing period (a.k.a. "next day pay"). |
delayed_filing | Untimely tax filing where delay is due to no fault of Check, including but not limited to re-filing (per filing). Only usage summaries exist for delayed_filing usage, and we will add usage records in the future. |
amended_tax_return | Each amended tax filing completed. Only usage summaries exist for amended_tax_return usage, and usage records will be added in the future. |
refile_attempt | Each new attempt to file a tax return on behalf of a company after a prior failure. Only usage summaries exist for refile_attempt usage, and usage records will be added in the future. |
mailed_tax_return_copy | Recipient copies of tax returns mailed to an employee. Only usage summaries exist for mailed_tax_return_copy usage, and usage records will be added in the future. |
Drilling into a usage category
Company com_dXE2p4wCM251HmlJB0Mn
generated its first wire to fund a payroll in June. To see all of its UsageRecord
objects representing funding wires, you can do so:
{
"previous": null,
"next": null,
"results": [
{
"category": "wire",
"company": "com_dXE2p4wCM251HmlJB0Mn",
"resource_type": "payment_attempt",
"resource": "pyt_vIFHhvyGN47ej1upyIT8",
"effective_at": "2024-06-01T00:00:00.000Z"
}
]
}
With this, you can tie the usage back to the specific PaymentAttempt
object and its corresponding payroll, and address any potential questions from com_dXE2p4wCM251HmlJB0Mn
. Note, effective_at
indicates when the "usage" was generated for the purpose of billing, and will be the first of the month in which the event occurred.
Resource Types
resource_type | Description |
---|---|
company | A Company resource. May be associated with the company category of UsageRecord . |
employee | An Employee resource. May be associated with the employee or one_day_payroll_processing_period category of UsageRecord . |
contractor | A Contractor resource. May be associated with the contractor or one_day_payroll_processing_period category of UsageRecord . |
payment_attempt | A PaymentAttempt resource. May be associated with the company_funding_failure , payee_failed_funding , or wire category of UsageRecord . |
Limitations and integration tips
Live data only. The Usage API only generates data from usage in the live environment. Sandbox will be populated with the mock data objects as outlined above.
Usage data only. Check uses underlying usage data to also bill you. Any negotiated aggregate fees or adjustments outside usage such as overall platform fees or credits will not be reflected in the Usage API.
Expect new usage categories over time. As Check releases new products or services, new categories may be added. Design your integration such that the existence of a new category
will not break functionality. Design partners for newer, usage-generating features may see these items on their bills not yet reflected in the Usage API. As of May 2024, usage data is not currently available for tax returns filed for terminated companies, Embedded Setup, Embedded Support, and product partnerships.
Usage is live up to a day prior and finalized after the end of the month. It is not safe to rely on this API to bill your customers for an active or upcoming period. Usage events will be generated throughout, and potentially unevenly due to the nature of when these usage events are generated. Ensure you wait until the following month to bill your customers. For example, bill for May in June. Finalized prior month data is expected to be available after 10:00am ET on the 1st of each month. May 1, 2024 is the earliest date for Usage data.
Resources indicated by resource IDs may not always exist. Resources that generated billable usage at one point in time may be deleted in Check later on due to your explicit deletion actions or authorized developer support actions taken by Check. If you encounter this, it means the resource existed in Check at some point, and you should still bill the associated customer.
Generating your platform's usage via API
To pull all the billable usage on your platform, you can generate the full list by paginating through the base /usage/summaries
endpoint for the period of interest. For example, in Python:
import requests
def fetch_all_summaries(url):
summaries = []
while url:
response = requests.get(url).json()
summaries.extend(response.get('results', []))
url = response.get('next')
return summaries
Downloading a report of your platform's usage via Console
To download all the billable usage on your platform, you can alternative generate the full list by logging into your Console, navigating to the Reports tab accessible to Admin
users, and selecting the month and usage report type. We will generate the report and email it to you once complete.
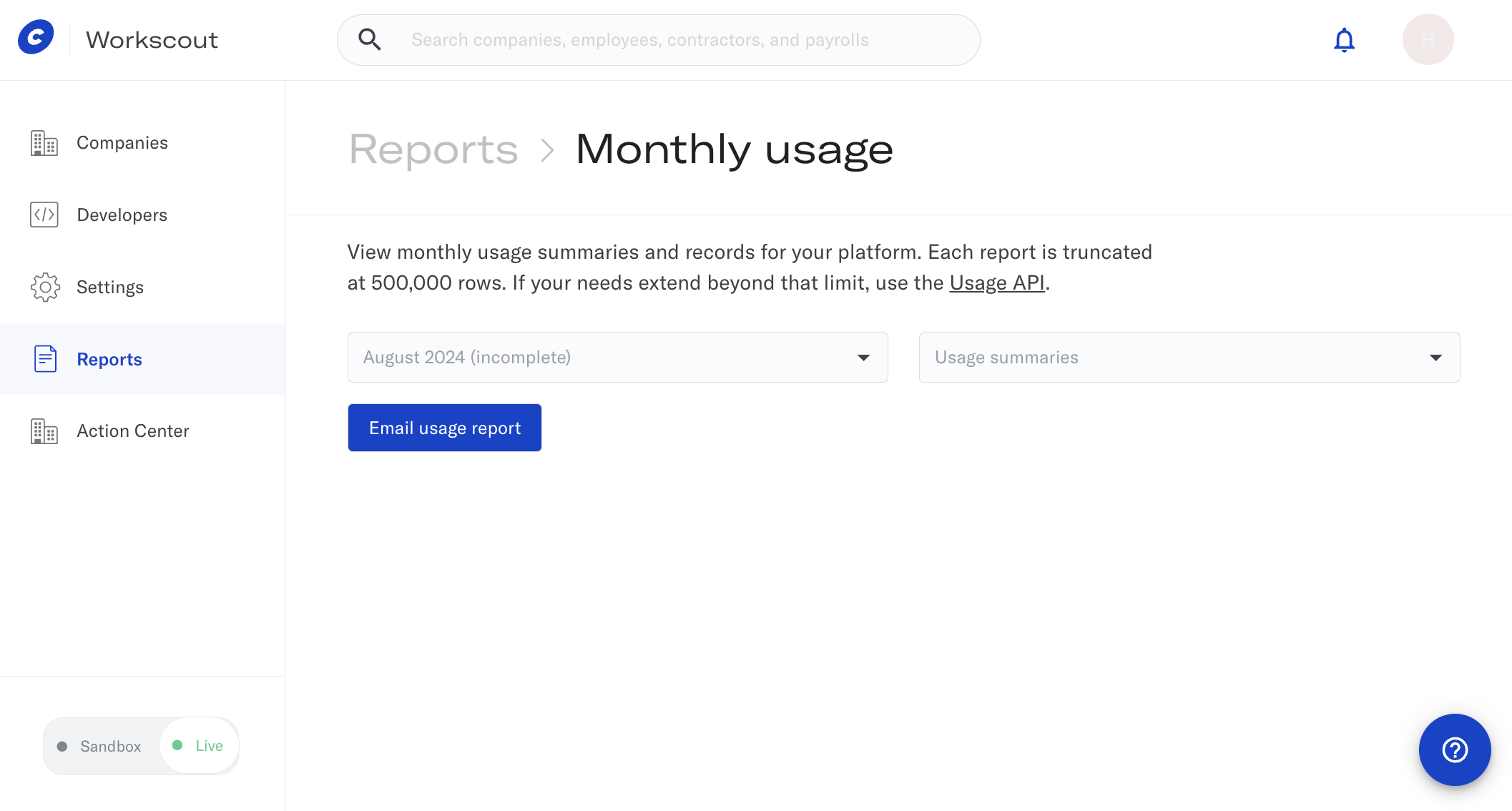
Summary
The Usage API enables you to more deeply understand and realize the full revenue of your growing payroll business. With it, you can confidently and efficiently generate clear bills for your customers and focus more on solving other important customer problems.
Updated 9 days ago